Getting Started with Richpanel
This page will help you get started with Richpanel. You'll be up and running in a jiffy!
What do you want to do with Richpanel API?
- Connect a Custom cart platform to Richpanel
- Read/Write/Update Conversation(ticket) data in Richpanel.
- Calling external apps from Richpanel (using HTTP targets)
Connect a Custom cart
NoteIf you are using Shopify, Magento, or WooCommerce, you don't need this API. Richpanel natively integrates with these platforms and you can connect them directly by navigating to Settings => Apps => Browse all integrations.
Glossary
- User: A customer or a visitor profile on Richpanel
- Events: Events are actions associated with a customer’s profile. Example: Events are an order placed by a customer, a fulfillment update on a customer’s order, a customer viewed a product, etc.
Step by step guide to integrate a custom cart platform
Get API Key
- Get API_KEY and API_SECRET from Richpanel Apps => Custom Connector.
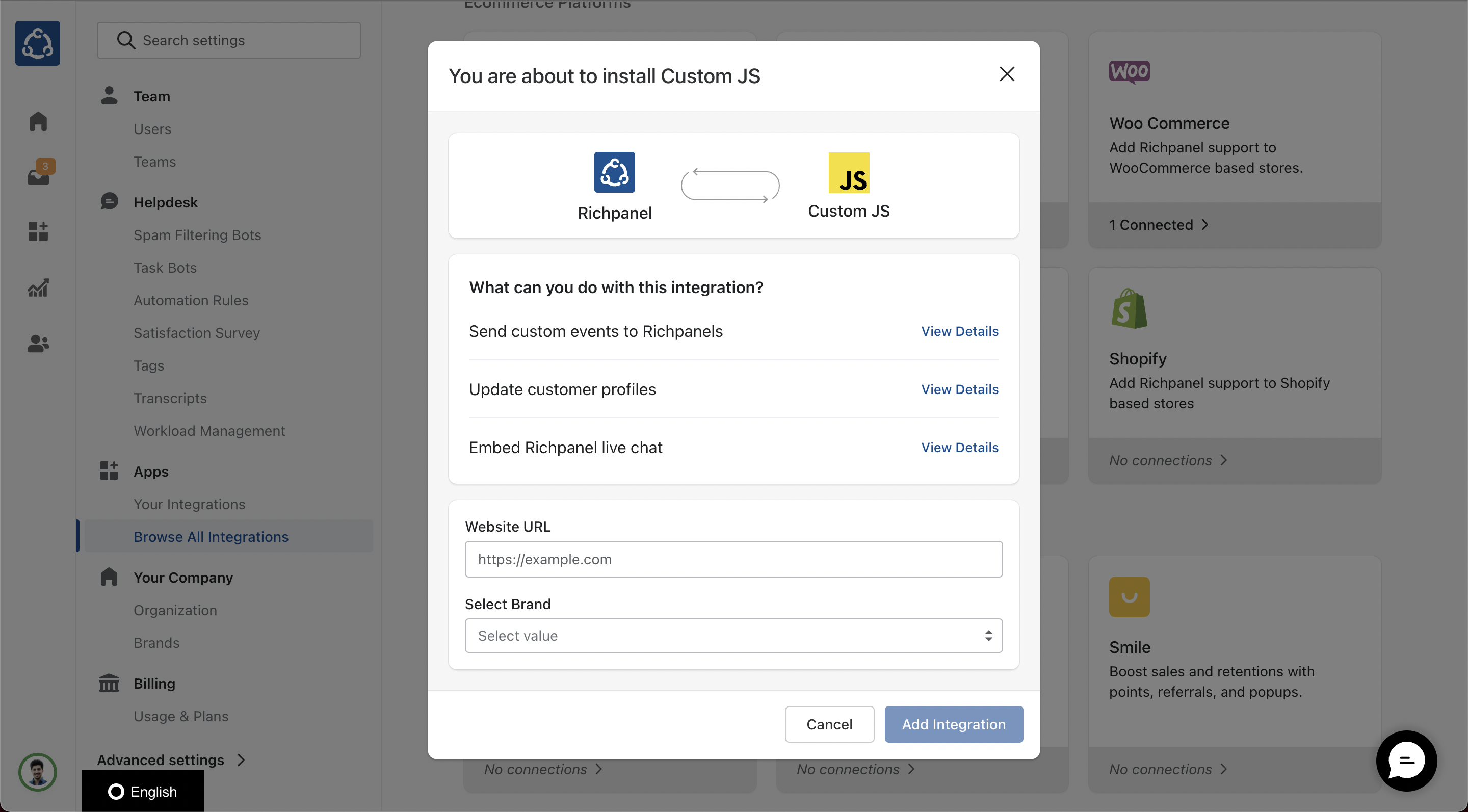
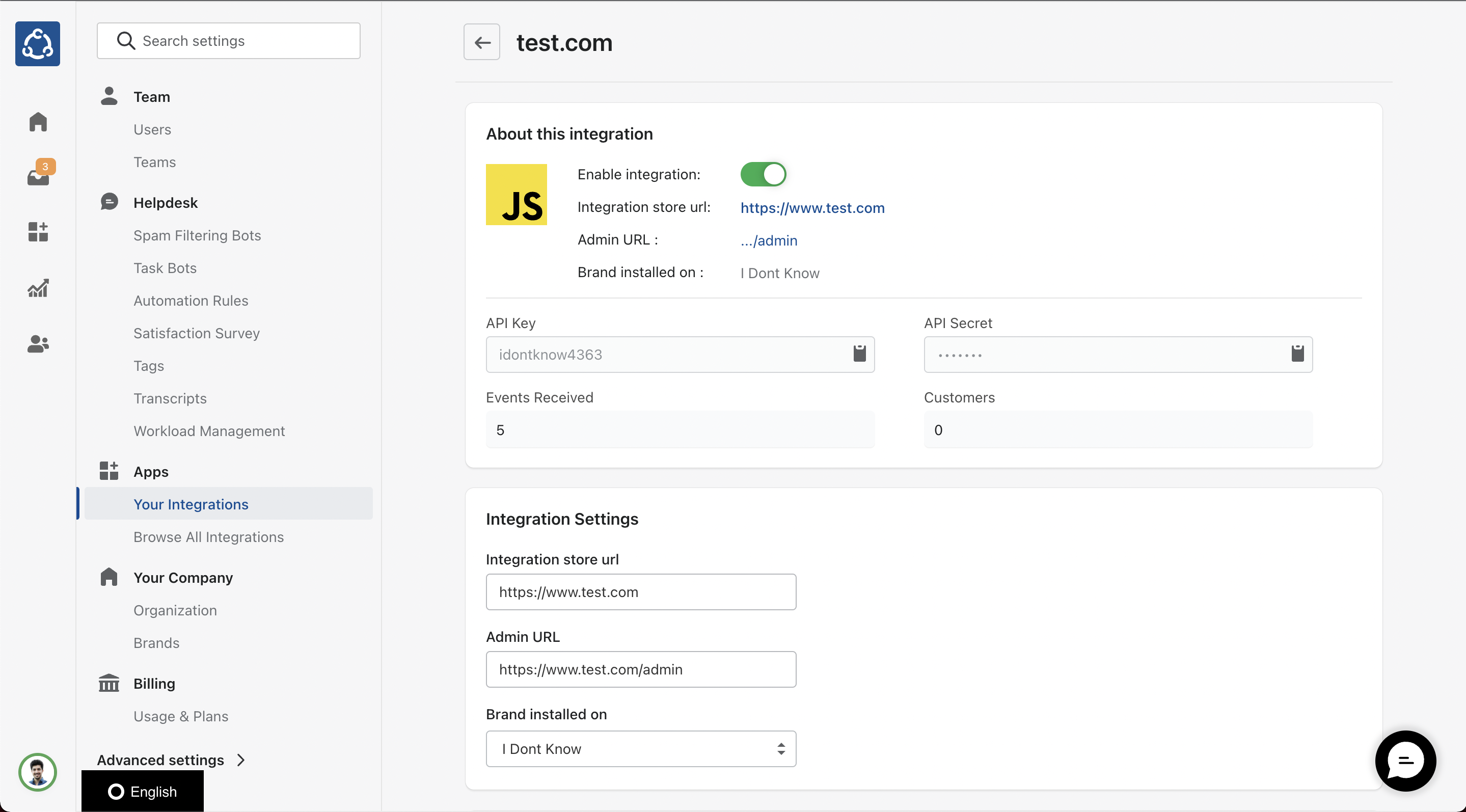
- Load the below script to a page where events need to be tracked.
window.richpanel||(window.richpanel=[]),window.richpanel.q=[],mth=["track","debug","atr"],sk=function(e){return function(){a=Array.prototype.slice.call(arguments);a.unshift(e);window.richpanel.q.push(a)}};for(var i=0;mth.length>i;i++){window.richpanel[mth[i]]=sk(mth[i])}window.richpanel.load=function(e){var t=document,n=t.getElementsByTagName("script")[0],r=t.createElement("script");r.type="text/javascript";r.async=true;r.src="https://api.richpanel.com/v2/j/"+e+"?version=2.0.0";n.parentNode.insertBefore(r,n)};
richpanel.load("API_KEY");
The above code will only load the Richpanel tracking script and Messenger (if enabled).
Identifying User and Authenticating them
Users can be identified using identify event, we track these users as visitors.
const userId = 'manojuid'
const properties = {
"firstName": "Manoj",
"lastName": "Patitapthi",
"city": "Manoj City",
"country": "Manoj Country",
"facebook": "manojfacebook",
"googleplus": "manojgoogle",
"instagram": "manojinsta",
"linkedin": "manojlinkedin",
"medium": "manojmedium",
"phone": 9977090122,
"uid": "manojuid",
"email": "[email protected]",
"billingAddress": {
"email": "[email protected]",
"firstName": "Manoj",
"city": "harlin"
}
}
richpanel.identify(userId, properties)
To convert them into customers, the platform must authenticate them. Follow the process below for Authentication:
- From the backend, generate the encrypted user data using API Key and API Secret
data = {
'id': '1234', // Required
'firstName': 'Adam',
'lastName': 'Smith',
'email': '[email protected]'
}
const crypto = require('crypto');
const encryptData = (data, secret) => {
const key = crypto.createHash("sha256").update(secret).digest('hex');
let iv = crypto.randomBytes(16);
let cipher = crypto.createCipheriv('aes-256-cbc', new Buffer(key).slice(0,32), iv);
let encrypted = cipher.update(JSON.stringify(data));
encrypted = Buffer.concat([encrypted, cipher.final()]);
return iv.toString('hex') + ':' + encrypted.toString('hex');
}
const encrypted_data = encryptData(data, API_SECRET);
- Pass that encrypted data in frontend and set it as
window.richpanelSettings
value.
window.richpanelSettings = <encrypted_data>;
Note: Make sure to load it before loading the Richpanel script.
Sending real-time events
To send an event, execute the below function to start tracking:
const event = 'order'
const properties = {
"orderId": "11111",
"orderDbKey": 2589211394106,
"email": "[email protected]",
"amount": 169.97, //required
"subTotalPrice": 159.97,
"totalWeight": 2812,
"weightUnit": "grams",
"taxAmount": 10,
"currency": "USD",
"status": "processing",
"discountAmount": 149.97,
"coupons": [
"code1"
],
"deleteFulfillmentAndCreate": true,
"statusUrl": "https://abc.com/10672582/orders/fd1051b534691f2cd00495d6f2fcdd45", //tracking url if any
"paymentStatus": "partially_refunded",
"fulfillmentStatus": "fulfilled",
"tipAmount": 0,
"orderName": "#606243",
"paymentMethod": "paypal",
"paymentGatewayNames": [
"paypal"
],
"processedAt": 1606758898000,
"processingMethod": "express",
"items": [{ //required
"id": "2493989519418",
"price": 49.99, //required
"optionId": "31858336956474",
"sku": "A31SSUJZ99COMTL1",
"name": "My Product - L",
"grams": 454,
"quantity": 1, //required
"imageURL": [
"https://cdn.abc.com/s/files/1/1067/2582/products/product1.jpg?v=1605207140"
]
},
{
"id": "4586759913530",
"price": 49.99,
"optionId": "32132068311098",
"sku": "A31SSUGI85ODGDM1",
"name": "My Product - M",
"grams": 454,
"quantity": 1,
"imageURL": [
"https://cdn.abc.com/s/files/1/1067/2582/products/product2.jpg?v=1605207140"
]
}
],
"billingPhone": null,
"billingEmail": "[email protected]",
"billingCity": "LOCKPORT",
"billingPostcode": "60441",
"billingState": "Illinois",
"billingCountry": "United States",
"billingAddressLine1": "201B, lotus CHS",
"billingAddressLine2": "",
"firstName": "Shubhanshu",
"lastName": "Chouhan",
"shippingPhone": null,
"shippingEmail": "[email protected]",
"shippingCity": "LOCKPORT",
"shippingPostcode": "60441",
"shippingState": "Illinois",
"shippingCountry": "United States",
"shippingAddressLine1": "201B, lotus CHS",
"shippingAddressLine2": "",
"shippingFirstName": "Shubhanshu",
"shippingLastName": "Chouhan",
"shippingMethod": "Free Shipping 2-5 Days",
"fulfillment": [{
"id": "2488098717754", //required
"createdAt": 1608611436000,
"updatedAt": 1609187662000,
"status": "delivered",
"quantity": 6,
"tracking": [{
"trackingNumber": "1234578162783",
"trackingCompany": "USPS",
"shippingDate": 1608611436000,
"trackingUrl": "https://tools.usps.com/go/TrackConfirmAction.action?tLabels=1234578162783"
}],
"items": [{ //required
"id": "2493989519418", //required, Product base id
"price": 49.99, //required
"optionId": "31858336956474", //product variant id
"sku": "A31SSUJZ99COMTL1",
"name": "My Product - L", //required
"grams": 454,
"quantity": 1, //required
},
{
"id": "4586759913530",
"price": 49.99,
"optionId": "32132068311098",
"sku": "A31SSUGI85ODGDM1",
"name": "My Product - M",
"grams": 454,
"quantity": 1,
}
]
}],
"shippingAmount": 10.45
}
richpanel.track(event, properties)
Follow this document to know further about events and structure.
Follow this document for order event structure detail.
Sending Bulk Events / Sending Order History
Old events can be sent in bulk to Richpanel, like order history.
To send batch events, the individual events should be arranged as follows:
const events = [{
event: 'order',
properties: {...orderProperties},
time: {
"sentAt": <order-date> //when event occurred, use this property in case you're updating old orders, or synchronizing old orders
},
appClientId: API_KEY
}]
const events = {
"event": "send_batch",
"appClientId": "test_api_key_123",
"events": [...events]
}
And further data can be passed to a batch API
const eventData = btoa(JSON.stringify(events))
const data = JSON.stringify({
"hs": eventData
});
const xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.addEventListener("readystatechange", function () {
if (this.readyState === this.DONE) {
console.log(this.responseText);
}
});
xhr.open("POST", "https://api.richpanel.com/v2/bt");
xhr.setRequestHeader("Content-Type", "application/json");
xhr.send(data);
Note: Always sync orders in the batch of 25 for smoother sync.
Updated 28 days ago